Article
How to Debug Django Apps
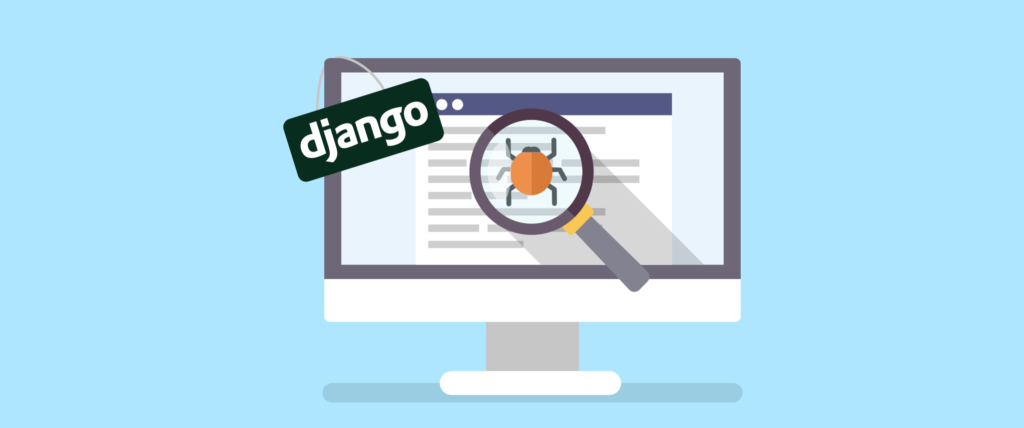
In the JavaScript world, my primary debugging workflow consists of dropping a debugger
or a console.log
into the code and seeing what happens in the browser’s developer console. This methodology has always worked effectively for me. However, I have started to work more with Python and Django recently, and though they do have similar debugging tools, it is not quite as simple under certain environments. In this article, I will go over the various scenarios I’ve come across and offer techniques for debugging a Django application.
print and pprint
console.log
is to JavaScript as print
is to Python. If you are using print to log out dictionaries, you might consider using pprint
instead, as it will nicely format your code with indentation.
from pprint import pprint; pprint(some_variable)
Yes, this is insanely verbose to type out every time, so I’d recommend creating a snippet for your editor.
pdb (The Python Debugger) and ipdb (IPython pdb)
pdb is like the JavaScript debugger. You can drop an import pdb; pdb.set_trace()
in your code and it will provide a REPL for you to step through your code.
ipdb is like an enhanced version pdb. Useful features include syntax highlighting and tab completion. It is enormously helpful to be able to inspect objects.
You’ll have to add ipdb to your requirements.txt and do the usual pip install -r requirements.txt
. And then you can add import ipdb; ipdb.set_trace()
anywhere in your code to use the interactive debugger.
Debugging Django in Docker
If you are using docker, you might notice that dropping a pdb or ipdb into your code does nothing. To fix this, you’ll have to run docker with service ports enabled. For example:
docker-compose -f docker-compose.yml run --rm --service-ports name_of_container
Debugging Django and Gunicorn setup in Vagrant
While pdb and ipdb work when you are using the default runserver command, once you move over to Gunicorn for development, you no longer get the code-stopping functionality. I still have yet to find a good solution to this problem.
However, I have discovered a better workaround for this. The answer lies in test-driven development. You can drop pdb or ipdb into your test cases as well as your application code. Then, when you run your test, you’ll get the code stopping functionality.
shell_plus
Another useful tool that I have found for debugging is shell_plus. You get this as part of the django-extensions package. This is useful when I need to experiment with various queries using the Django ORM.
Conclusion
I hope you found this information helpful and hope you saved some time figuring out why the “Python debuggers” aren’t working.