Article
Optimizing Laravel Performance
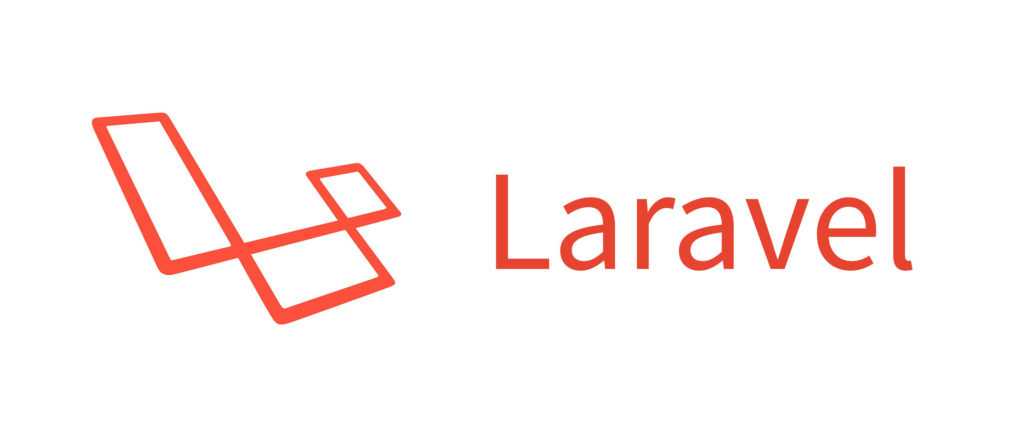
Laravel is currently the most popular PHP framework for building a variety of sites and applications. Here at Fresh, we’ve built both complex Laravel API platforms and simple portals. The set of libraries and architecture that Laravel provides makes it easy to build amazing sites using well structured and “beautiful code” (their words exactly).
Sometimes with frameworks you wind up sacrificing performance for ease of use. Laravel has done a good job at keeping their framework performant, but here are a few things you can do to help further increase Laravel performance.
Minimize Plugins
There are a wide variety of plugins for Laravel that allow you to easily add more functionality. With that increased functionality come more libraries (and files) to load, which can slow things down. Make sure to take a peek at which providers you are loading through your config/app.php file and trim out unnecessary ones. In addition, Laravel uses composer to manage its components, so pruning your composer.json file will help reduce the dependencies that are loading.
Use “Eager Loading” of Your Data
Laravel uses the Eloquent ORM to easily map your object models to the database tables supporting it. With a few simple files you can map out your object structure, and Eloquent will handle all the appropriate database interaction needed for your CRUD (create, retrieve, update, delete) operations. When Eloquent does it, it uses a “lazy loading” approach. That means for any related data, such as the author details for a book, Eloquent won’t actually retrieve the data until it is specifically referenced somewhere else in the code. While it may seem harmless to have one or two extra queries on your details page, making a similar query against a collection of books can result in a lot of queries and reduced performance as you wait for all of your data to return.
Instead, you want to set up your queries to use “eager loading,” which means they will retrieve any associated object models as part of your initial query. That way they are immediately available for use. Your typical…
[code]
$books = AppBook::all();
foreach ($books as $book) {
echo $book->author->name;
}
[/code]
…lazy loaded query becomes an…
[code]
$books = AppBook::with(‘author’)->get();
foreach ($books as $book) {
echo $book->author->name;
}
[/code]
…eager loaded query.
Laravel provides a nice overview on this here.
Trim Your Response Data
This typically applies to API type frameworks, but can also apply to traditional MVC applications created within Laravel. With Eloquent at your fingertips, it is easy to query a comprehensive set of data to be used for display. For example: your book may have a collection of authors, and each of those authors has a basic biography, which also includes a list of events (and their related locations) that they are appearing at over the next three months. When you return a large list of books, regardless of how fast the query completes, passing all of this related data increases the data return size, and causes a delay as the client waits for the data.
If you don’t need all of this data, then you’ve wasted a lot of time (and bandwidth) in your app. The solution to this is to use “View Models,” an optimized subset of data from your data models, containing only the data elements you need for display. If you’re building an API or returning JSON data, you can use a library such as Fractal to easily translate your object model data and include only what you need.
Precompile Assets
For development, having all of your assets in separate files (such as routes and configuration files) is helpful for code maintenance. For production, this isn’t necessary. To help with this, Laravel has a few artisan commands available that you can run before deploying your site:
[code]
php artisan optimize
php artisan config:cache
php artisan route:cache
[/code]
These commands will compile your frequently used classes into a single file for quick reference. They will also combine your configuration files and routes into single files for faster loading. You can also add your own classes into the optimization that might not be added by default. The performance increase will vary, but every little bit helps. More details about these optimizations can be found here and here.
Consider Lumen
Are you building an API or a really simple application that doesn’t require a “full stack” framework? If so, consider Lumen, a micro-framework that has the same elegance in mind as Laravel, just without a lot of additional libraries you may not need. Lumen is stateless, so complicated authentication and session management is replaced to leverage API tokens or headers.
Conclusion
Laravel provides a great framework and good performance out of the box. As your code grows more complex, you may need to optimize a few things for increased performance, and these tricks should help you along the way.