Article
Dev Principle #2: Coding Layout and Style Matter
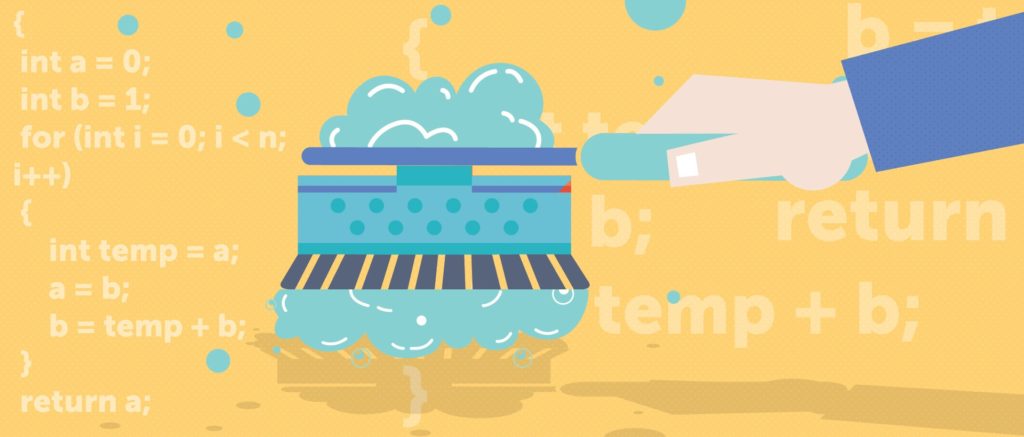
We’ve all seen it before: terribly formatted code.
No layout or style. Unindented, with insanely long line lengths. Tabs and spaces cobbled together haphazardly. Multiple inconsistencies along a single code file. Code formatting has gotten so bad that famous software webcomics have commented on the issue.
The point is, code should be easily scannable. Other software developers should be able to determine what is going on, logically, in just a few seconds. Clean code enables easier collaboration between internal and client developers, improved productivity, and easier debugging, among other benefits.
To avoid letting your code deteriorate into hieroglyphic chicken-scratch, here are a few tips to keep it clean, legible, and uniform.
Keep It Clean
Start off by keeping your variable declarations outside of the logic.
Instead of:
function foo() {
int x;
int y;
if (...) {
y = ...;
}
int z;
if (...) {
z = ...;
}
return x * y * z;
}
Try to keep it formatted like this:
function foo() {
int x, y, z;
if (...) {
y = ...;
}
if (...) {
z = ...;
}
return x * y * z;
}
In most scenarios, it’s best to declare variables at the top of a function – these declarations should include statements at the top of the code file. Cleaning up your code in this way will allow people to easily scan, seeing what variables exist and how they are used.
Keep your functions short and keep them in a logical order. When creating functions, the name should describe exactly what that function does.
Instead of:
function gcoli(x) {
return color[x];
}
Try this:
function getColorByIndex(inputNum) {
return color[inputNum];
}
If you look at the function – both where it is declared and where it is used – you immediately know what it does.
Additionally, the functions in the file should be organized logically. This helps other engineers scan through the code and better understand what is going on.
Don’t be afraid to use whitespace. Code often benefits from whitespace between major sections. In fact, many coding style guides call for functions between methods and classes, or space between brackets and after commas.
Consider:
$().doSomething(function(){
// do stuff here
},‘parameter’,2,3,2);
$().doSomethingElse(function(){
// do other stuff here
},‘parameter’);
The previous snippet looks messy to the eye. A bit of whitespace can clean this up:
$().doSomething( function() {
// do stuff here
}, ‘parameter’, 2, 3, 2);
$().doSomethingElse( function() {
// do other stuff here
}, ‘parameter’);
Keep It Legible
Code should be easy to read. To make your code easier to read, indent it in a way that makes sense for the language and for other users. Proper indentation will allow others to easily notice where code blocks start and end.
Different programming languages and frameworks have style guides stating how many tabs or spaces should be used for each code block. Software Monkey’s article on this subject shows many great examples.
Also, do your best to keep lines below a specific set width. This saves the reader from having to scroll to the side or trying to figure out which lines soft-wrap and which ones don’t. Most older computers had a screen/terminal character width of 80 characters wide, which is why older style guides such as the infamous PEP8 state 79 characters as the maximum width of a single line.
In modern machines, we have the benefit of much longer line widths – sometimes upwards of 300 characters in a single screen! The middle-ground lies at around 119 characters, which is the contemporary standard terminal or console size.
Most editors have features or tools that show a vertical line at the 79 and the 119 character marks so that you can more easily keep track of the line widths and remember to keep lines short.
Aside from forcing the reader to scroll to the right, having a line longer than 119 characters is usually a sign that the line could be broken up into smaller lines, or that the variables in that line are not named well – check out Dev Principle #1: Choose Appropriate Variable Names to read more on this subject.
Keep It Uniform
Whatever indentation method, whitespace pattern, or style guide you have chosen for your project, it’s very important to enforce that style going forward. It doesn’t need to be you or another human keeping a keen eye out for these styles when someone makes an edit. There are “linters” and other automated tasks that will check this for you at different parts of the coding process. From saving the file to making a commit, from making a pull request to deployment and production, these automated tasks keep the project uniform.
It’s an essential best practice – good coding layout and style allow the project to be easily produced, upgraded, and maintained through its entire lifecycle. If the code document has been edited by someone other than the original author, the edits should be so uniform that the edited lines are indistinguishable. This topic is expanded upon in “The Boy Scout Rule.”
There are many great examples of clean code out on the web – for an example on what not to do, check out the “Just another Perl hacker” challenge.
Conclusion
Moving forward, we recommend keeping your code clean, legible, and uniform throughout. The suggestions above are a fine place to get started.