Article
10 Development Tips for Building a Solid Foundation
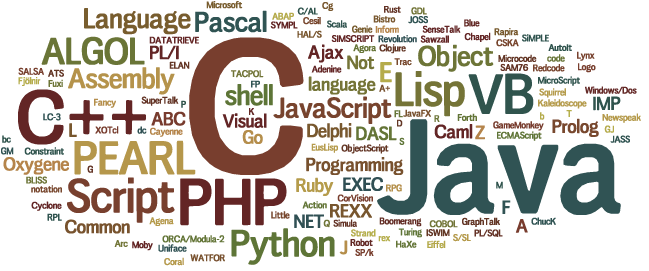
Being a developer these days is both easier and harder than it used to be. We have the benefit of “standing of the shoulders of giants” and leverage a lot of technology that wasn’t there before. Imagine trying to build Facebook using nothing but Assembly language! However, we also live in a far more complex computing environment than we have in the past, with various platforms (mobile, tablet, desktop/laptop), online vs. offline concerns, security issues, and more to keep in mind.
Establishing a solid foundation for your development environment can really help you accomplish your projects quickly, as well as decrease the frustration and confusion that can occur along the way. Here are some tips and best practices that you can use in your own development environment.
1. Pick the right tool for the right job.
Just because the last three apps you wrote used .Net does not mean your fourth has to use it as well. Case in point: A few months ago I volunteered to renovate a really old site for a church. They were using Front Page/HTML and the person in charge was overwhelmed on how to maintain it and issues were occurring. My first though was to set up a CMS for them, and I picked an open sourced, .Net one with a lot of good reviews, that was perfect for medium/large organizations. I spent a week of dealing with hosting incompatibilities, learning a new CMS, and structuring a lot of templates and controls without much progress to show for it.
Then I took a step back and thought about things more. I realized that WordPress better suited their needs (simple page content with a feed for their blogs and sermon messages) was a lot easier to get running, and was a lot easier for them to update their posts with. I had things installed and running within the day and the rest of the content imported within a week. The right tool fit the right job and made everything a lot smoother to implement.
2. Don’t Reinvent the Wheel.
I’ve run into countless scenarios where the first decision that is made is to build system X without seeing if there is already an alternative out there. I’ve seen CMS systems built from scratch, often with fewer features and more bugs than something freely available. You have CMS systems like WordPress, PyroCMS, Joomla, Orchard, and Umbraco. You have languages such as .Net, Ruby on Rails, Python, Haskell, and F# to get sites and applications running. Even within those you have frameworks like MVC, MVVM, CodeIgniter, jQuery Mobile, Entity Framework, PDO, and DataMapper that help your application get developed even faster. My rule of thumb when approached with a new project is to gather the requirements and see what language/framework fits best to get the project up and running (see, I’m following my own point #1!!) If nothing fits well (which happens on occasion) then it is time to build something up from scratch, but I’d wager to say that it happens only 5% of the time. See the list of various programming languages.
3. Coding standards aren’t king. Consistency is.
I’ve been coding for about 10 years now professionally and I’ve seen nearly every type of coding standard (Pascal, Hungarian, Camel, Homegrown) there can be. Often enough you’ll run into people that have some REALLY strong opinions on the “right” coding standard. They will reach the point to which they will argue, rather vehemently, about their standard as the “one and perfect” standard by which all others fail. The important point is that coding standards were put in place to provide a consistent way to quickly analyze the code you are working. Being able to quickly analyze your variables and controls, so that you can see where a particular object might be giving you grief, is the ultimate objective. Having this consistency also helps when you bring a teammate on to help you with some code, because they can understand what the code is attempting to do without having to relearn everything. Ultimately it doesn’t matter WHAT standard you pick, as long as it is something everybody in your team adheres to and understands. See Language specific naming conventions to learn more.
4. Just like voting, document and communicate early and often.
Even when you have two seasoned programmers on the same team. There can be plenty of confusion as to what a given method is doing or why. More importantly, there’s a lot of miscommunication that can occur between the customer, the marketing team, and the programmers themselves when it comes to the details of a project, as outlined here. It’s key to keep good documentation of your application and the customers needs for future maintenance.
Keep pertinent “why” type comments in the code for a given loop or calculation. Keep a Wiki or other online documentation source to outline higher level structural organizations for a project. Use the comment system in your source control system to document changes to various points in code (bug fixes and enhancements). Most importantly, keep good phone or e-mail contact with your customer to make sure their needs are being met. I’ll admit that translating the “geek speak” to “business speak” can be the hardest barrier to overcome, but it is worth it when your customer feels confident that their needs are being met. Often I find having the customer outline their use case scenarios (I need to click button X and have it bring me a list of Y’s) work best. From there you can look at their use case scenarios and identify key data points (Y’s reside in table A and B) and business rules (we don’t pull records from table C because that is only for expired records).
5. Sanitize your data input and output.
Even with all the advanced frameworks and languages out there today, SQL Injection, XSS hijacking, and even simple HTML character escaping cause a lot of headaches on websites today. Some frameworks will do a good job at parameterizing your SQL queries, but some will not. It is important to look at your given framework and make sure to discover this and do the appropriate code modifications as needed. Every language/framework you work with will have a document on how to properly handle your database queries.
While it used to be common to trust your data coming out of the database, even your output can come under attack. One tactic of the ASPRox botnet virus was to attempt to read the system tables on a database, and thus gain access to all the database tables installed. In hosting scenarios where multiple sites were using the same database, site A may have been infected, but the virus spread itself through the database, so that site B was attempting to affect visitors to their site, because their tables had been corrupted too. Most languages provide an “escape HTML” type function that you can use to sanitize your output. See Bobby Tables for the illustration of problem.
6. Source control is your partner
It’s hard to think of a day when I WASN’T using source control, even for small side projects. When I was, I cringe in fear at what would happen if something happened to my primary machine where the code resided. Source control allows you a way to easily back up your code and even more importantly, be able to track your changes between updates and features added to your project. While source control might not seem necessary if you’re working by yourself on a project, the second another person is brought on to the project, or somebody else is to take over for you, source control proves it’s weight in gold. Source control proves even more valuable when you’re working in a telecommute environment or with colleagues that may be half way around the world. Source control will give you a great way to see the fine details of what has changed between various versions of your code, which can also help debug issues that have been introduced during a code change. There are lots of free source control options available, most notably SVN, git, and Mercurial. There are also paid systems like Vault and Team Foundation Server. Most bug tracking and ticket systems will also integrate with these source control systems, so you can link up your code changes with their relevant work items.
7. Personal organization and workload is important.
When you’re working on more than one project at the same time, or you have multiple requests getting thrown at your daily, keeping yourself organized becomes a priority. It is also important not to overload yourself with work. Jim Benson make a great observation that you can’t work at 100% capacity because you can’t effectively switch between your tasks to get them done. The trick is to find the balance of tasks that you can do simultaneously without losing your throughput. You also need to find a way to keep these tasks organized, as well as all the other ones that are waiting to be done.
A couple of years ago I stumbled upon something called the Personal Kanban. It’s modeled after the larger scale agile programming tool called the kanban board. It is a great way for you to organize your tasks, track the status of them (working on vs. complete vs. waiting to start). It also helps you limit your workload so that you don’t reach that 100% limit. This approach has really increased my productivity, because I keep myself from being overloaded with work and I can easily switch between tasks if something comes up that is more important.
8. Take time out to learn.
“Never stop learning” is the motto they use in a lot of places and I firmly believe it. It’s easy to become completely consumed with the work you have for the day, that by the end of it you’re too tired to do anything else when you get home. Days turn into months, and months turn into years before you know it. Suddenly, everybody is talking about “Hot Tech Gamma” that has been out for a year now, generating a lot of hype, and you have no clue what it is. This hurts your personal development as a developer, as well as hurts the company you work for. I consider it my duty to bring fresh options to my boss for consideration when a new project comes up, and you should too.
My recommendation is to take a minimum of 3 hours out of your work week to research new technologies or even new tech news. You can do this all at once, or take roughly 30 minutes a day to read up on your favorite tech blogs or news outlets. You could even do something crazy like browse through questions on StackOverflow that intrigue you and learn things that way. If you’re working a typical 40 hours a week, this small investment will pay off big down the road when a problem arises or project comes up and you say, “Hey, I remember reading up about something last week that would fit perfectly for this!”
9. Have a hobby project
Sometimes the best way to learn is by doing. While you are writing a lot of code at your job, you might not get a chance to look into those new technologies that you’ve been reading about, because they’re too new, or there’s not enough known about them to implement in a major project. In addition, the more you code, the more you learn about your programming language, even if it’s the subtleties of the language. This is where a hobby project comes in handy. Finding a side project (like building an online database for your favorite collectible card game) gives you the opportunity to try out new technologies without the worry, limitations, or deadlines of your current job. You’ll also have an added enthusiasm to complete the project that you might not have with your current project at work.
There are also lots of open source sites that encourage contributions from the public, like GitHub, CodePlex, and Sourceforge. You can easily look through these sites for a project that excites you and start contributing. Plus you can add a little geek cred to your resume when you say “I helped update that feature” or “That’s my project I put out there for everybody to use.” More importantly, you’ll get more exposure to the development process you love to do.
10. Share the love, start a blog.
Finally, share your knowledge with other developers that are just starting or looking for answers. You can also use your blog to document those odd solutions (such as computing the haversine formula in your database) to which you may want to refer to later. More importantly, just as you’ve spent your time online, reading other blogs and resources to learn, you can pass that knowledge along to others that stumble across your blog. I was told once that the best way you can tell that you understand the code you’re writing is if you can explain it to others. The blog provides a great medium for you to do that as well. Take your existing code, or maybe some other code you found out on the Internet, and do more than just show how it works, but show why it works as well. Maybe there’s a clever trick in its calculations, or it fits a specific need by a customer. Being able to outline those things in a way that other developers can understand makes you a better developer as well.
Hopefully these tips inspire you to set a new (or rebooted) environment in which to develop in. Notice that a lot of these principles are interrelated. You could start your own blog, have a hobby project of creating some plugins for it, share those plugins on a code repository, and then document in your blog how and why they work. The knowledge you gained from those plugins might help a couple months down the road when your business wants to take the “social media” plunge and get a blog going on their site, with some custom features to it that your plugin would solve perfectly. In the end will make you a better software craftsman, and you (and your employer) will be better for it.